We have found out that there are nuanced distinctions between C and C++, from their syntactical intricacies to performance implications. Let's see what those are.
More...
If you're starting your journey in programming, you'll soon realize that choosing a programming language can be a daunting task. The programming language you choose can have a significant impact on your coding journey, affecting everything from your job prospects to the types of projects you can build.
We take a closer look at two popular programming languages, C vs C++. We'll examine the differences, strengths, and practical uses of both languages to help you make an informed decision on which language to use for your coding projects.
Key Takeaways
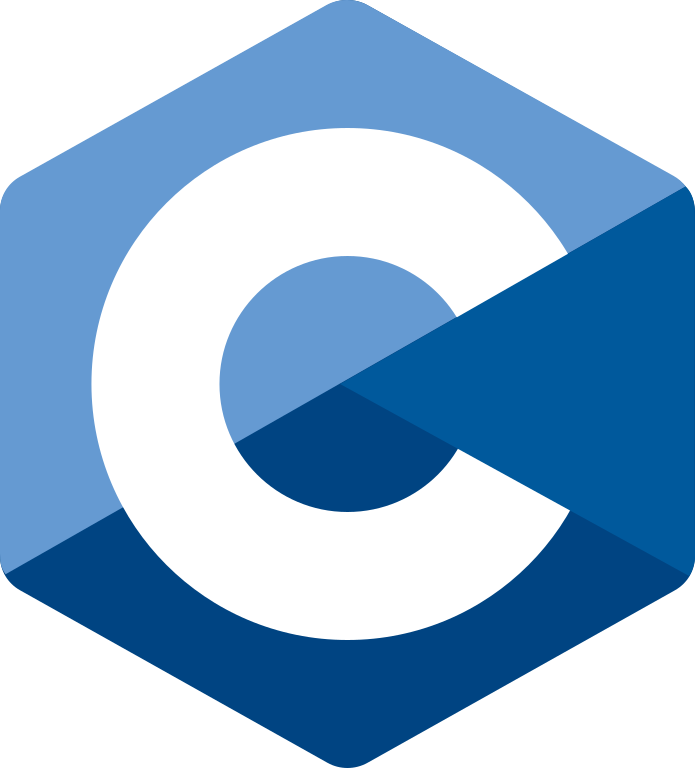
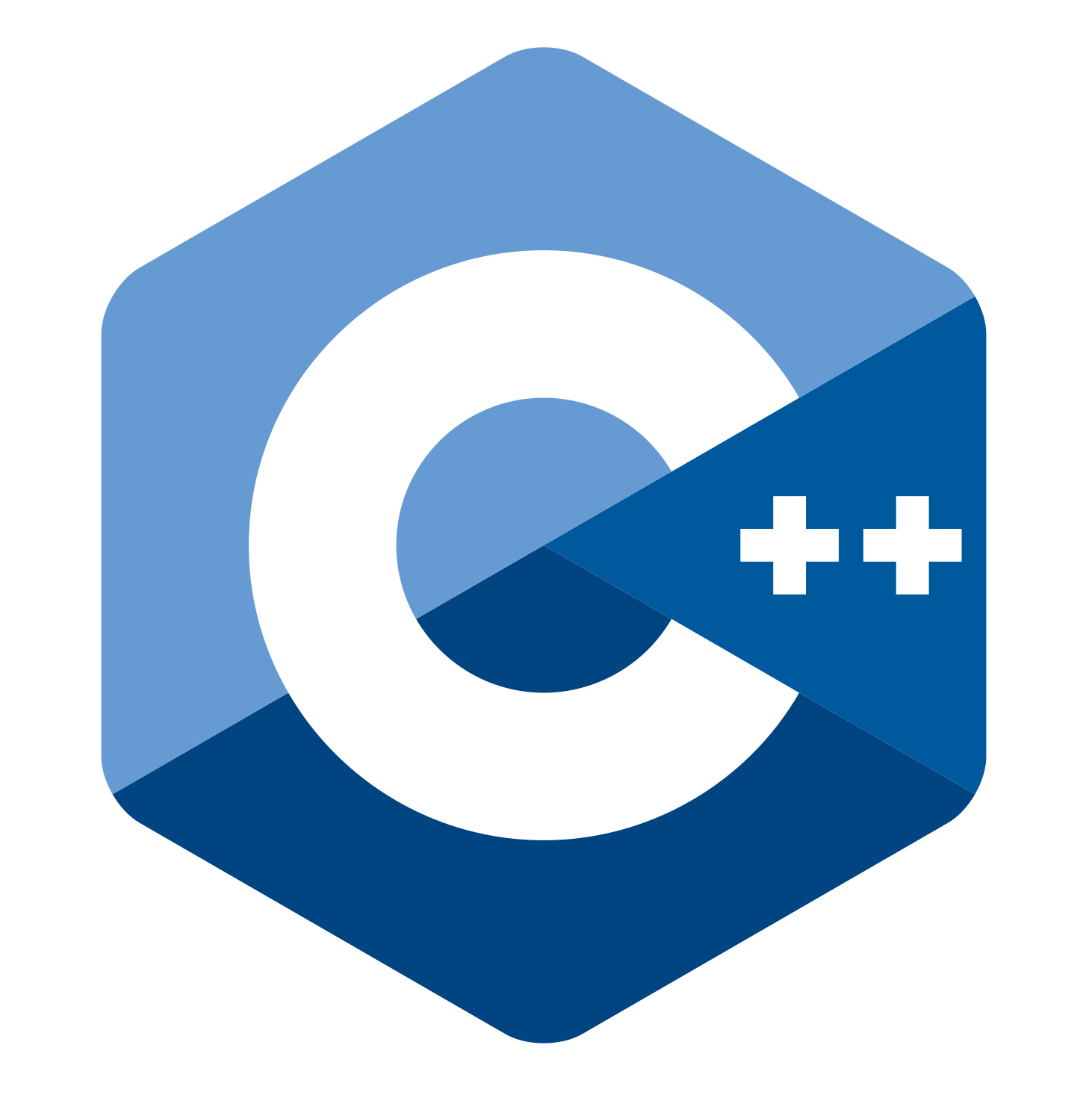
Introduction to C vs C++
At the core of programming languages, you'll find C and C++.
C is a high-level programming language known for its simplicity and efficiency, while C++ is an extension of C that adds object-oriented programming (OOP) features. Together, they form the C family of programming languages.
Learning C and C++ can open doors to a wide range of programming opportunities, as they are used globally in various industries.
The Significance of C vs C++
Although C and C++ were created decades ago, they remain relevant today. They form the foundation of numerous operating systems, including Unix, Linux, and Microsoft Windows. C and C++ are also used in system programming, embedded systems, game development, scientific and numerical computing, and more.
How They Work
C and C++ are compiled languages, meaning the code you write must first be translated into machine code by a compiler before it is executed. This process ensures that the code runs efficiently on the target platform.
Both languages are powerful and efficient, but C++ adds an additional layer of complexity with its OOP features. However, knowing C++ allows you to take advantage of OOP concepts such as encapsulation, inheritance, and polymorphism to write more organized and modular code.
Code examples for printing:
- 1C: printf("Hello, World!\n");
- 2C++: std::cout << "Hello, World!" << std::endl;
Syntax Comparison
The syntax of a programming language refers to its grammar and structure. It determines how code is written and how statements are executed. In this section, we explore the syntax of C and C++ and highlight their similarities and differences.
Data Types
Both C and C++ support basic data types such as integers, floating-point numbers, and characters. However, C++ extends this by introducing new data types such as boolean, string, and user-defined types.
C Data Types:
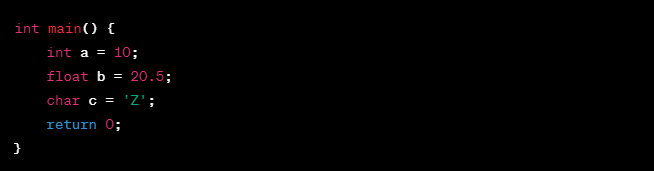
C++ Additional Types:
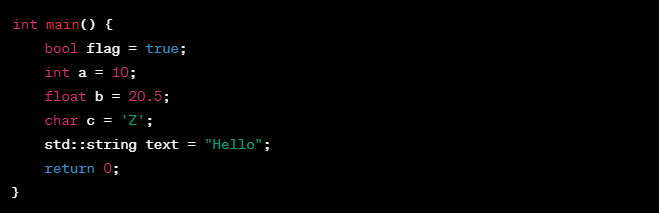
Control Flow
Control flow refers to the order and manner in which statements are executed in a program. C and C++ share the same control flow statements such as if-else, for, while, and do-while loops.
Functions
Functions in C and C++ are defined using the same syntax. However, C++ supports function overloading, which means you can define multiple functions with the same name but different parameters. This allows for greater flexibility and reusability of code.
C Function:

C++ Function Overloading:
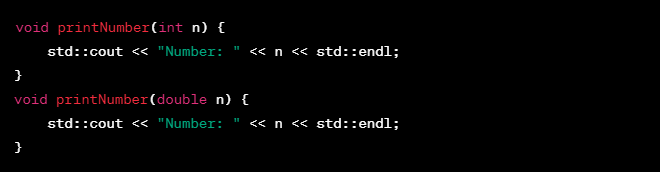
Pointers
Pointers are variables that store memory addresses. Both C and C++ support the use of pointers, but C++ provides more features such as reference variables, which are aliases for existing variables, making it easier to work with pointers.
C Pointers:
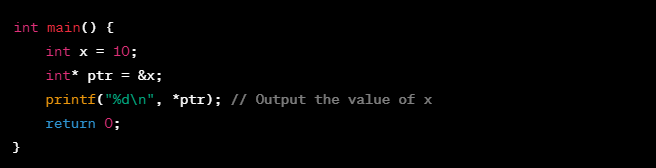
C++ References:
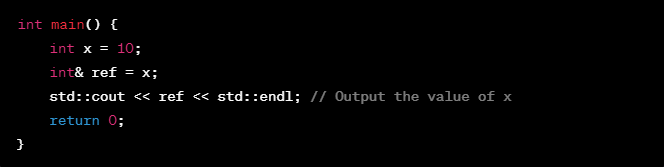
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that allows for the creation of objects that contain both data and behavior. C++ is an object-oriented programming language, and it provides features such as classes, inheritance, and polymorphism that are not available in C.
Memory Management and Pointers
Memory management and the use of pointers are fundamental aspects of programming in both C and C++. However, the way these languages handle memory management and pointers is quite different. Here, we explore the similarities and differences between C and C++ in these areas.
Memory Management in C and C++
In C, memory management is primarily done manually using functions such as malloc and free. This means that the programmer must keep track of the memory allocated to each variable and release it once it is no longer needed. While this offers more control and allows for efficient memory usage, it can also lead to memory leaks and memory errors if not handled correctly.
C Memory Management:

C++, on the other hand, has automatic memory management through the use of constructors and destructors. When an object is created, C++ automatically allocates memory for it, and when the object is no longer needed, C++ deallocates the memory. This prevents memory leaks and makes memory management much easier for the programmer.
Pointers in C++
Pointers are variables that store memory addresses. In C, pointers play a vital role in memory allocation and manipulation. However, they can also cause issues if not used correctly, such as memory leaks and segmentation faults.
C++ also uses pointers, but it includes additional features such as references and smart pointers. References are similar to pointers but are safer to use, as they cannot be null and do not require special syntax for accessing their values. Smart pointers, such as unique_ptr and shared_ptr, are objects that manage memory automatically, reducing the risk of memory leaks.
C++ Smart Pointers:

Comparison
Feature | C | C++ |
---|---|---|
Manual or Automatic Memory Management | Manual | Automatic |
Pointers | Used extensively | Enhanced with references and smart pointers |
Object-Oriented Programming (OOP) of C vs C++
C++ introduced the concept of Object-Oriented Programming (OOP) to the C language. OOP is a programming paradigm that focuses on the use of objects that contain data in the form of attributes and methods that operate on this data.
In C++, classes are used to create objects, encapsulating data and methods into a single unit. This provides a more organized and modular approach to programming, making it easier to manage large codebases. Additionally, C++ allows for multiple inheritance, where a class can inherit from multiple parent classes, increasing code reuse and flexibility.
In contrast, C is a procedural language that does not support OOP natively. However, it is still possible to implement some OOP concepts in C using structs and function pointers to emulate classes and methods, respectively. This approach is not as efficient or intuitive as the one offered by C++.
C vs C++ OOP Comparison Table
Aspect | C | C++ |
---|---|---|
Inheritance | No support for inheritance | Supports multiple inheritance |
Encapsulation | Can be achieved using structs and function pointers | Native support through classes and access specifiers |
Polymorphism | Cannot achieve polymorphism without using function pointers | Native support through virtual functions and interfaces |
The table above summarizes the major differences between C and C++ in terms of OOP support. As shown, C++ offers better support for OOP concepts, making it a more flexible and powerful language for developing object-oriented applications.
Standard Libraries of C vs C++
Standard libraries are a crucial aspect of any programming language. They provide developers with pre-defined functions and classes that can be used to perform common tasks. In this section, we explore the differences between the standard libraries of C and C++.
C comes with a small standard library that provides basic functionality, such as input/output operations and string handling. On the other hand, C++ has a much larger standard library that includes functionality for object-oriented programming, data structures, and algorithms.
C String Manipulation:

The C++ standard library is divided into several categories, including:
Category | Description |
---|---|
Input/Output | Provides functionality for reading and writing data |
Strings | Includes functions for manipulating strings |
Containers | Provides classes for managing collections of objects |
Algorithms | Includes functions for performing common algorithms, such as sorting |
C++ also includes the Standard Template Library (STL), which is a collection of generic classes and functions that can be used with any data type. The STL contains containers, algorithms, and iterators that simplify complex programming tasks.
C++ STL String Manipulation:

Overall, the C++ standard library is much more comprehensive than the standard library of C. However, both languages have their strengths and weaknesses in terms of library support.
Performance and Efficiency
Performance and efficiency are crucial factors to consider when choosing a programming language. While C is known for its speed and efficiency, C++ takes it a step further by introducing new features like inline functions, templates, and operator overloading. These features can help optimize code execution further, resulting in faster and more efficient programs.
Additionally, C and C++ allow direct memory manipulation, giving them an edge in performance compared to managed languages like Java or Python. However, this also means that memory errors and leaks can occur if not handled correctly.
Language | Performance Characteristics |
---|---|
C | Compiled code executes quickly and efficiently. |
C++ | Faster than C due to features like inline functions, templates, and operator overloading. |
Containers | Provides classes for managing collections of objects |
Algorithms | Includes functions for performing common algorithms, such as sorting |
Both languages have been implemented in various high-performance software, including operating systems, embedded systems, and game engines. Therefore, the choice between C and C++ ultimately depends on the specific requirements and constraints of the project.
Portability and Compatibility
One of the significant differences between C and C++ is their portability and compatibility.
C Portability
C is a portable language, meaning it can be compiled and executed on different platforms without making any modifications to the code. Developers can write C code on one platform and compile it on another platform without any issues. This makes C an efficient language for system programming and cross-platform development.
However, C does not offer a standard library for handling graphics, user interfaces, and other high-level functionalities. Developers must rely on external libraries to fulfill these requirements. It also lacks the object-oriented programming (OOP) paradigm offered by C++.
C++ Portability
C++ inherits the portability of C and offers additional features, making it more versatile. C++ code can be compiled and executed on different platforms without any platform-specific modifications.
C++ provides a standard library that offers functionalities such as input-output operations, string manipulation, and file handling, among others. The standard library also includes a set of container classes and algorithms that simplify development.
Furthermore, C++ offers OOP support, making it an ideal choice for developing complex software systems. The OOP paradigm enhances code reusability, flexibility, and maintainability.
Compatibility Comparison
When it comes to compatibility, C and C++ share some similarities. C++ is an extension of C and is backward compatible. This means that C code can be compiled and executed using a C++ compiler.
However, the reverse is not true. C compilers cannot interpret C++ code. C++ introduces new keywords, classes, and features that are absent in C, making it incompatible with C compilers.
C | C++ | |
---|---|---|
Portability | Portable | Portable with additional features |
Standard Library | Lacks high-level functionalities | Offers a comprehensive standard library |
Object-Oriented Programming | Does not support OOP | Offers robust OOP support |
Compatibility | Can be interpreted by C++ compilers | Incompatible with C compilers |
Industry Applications when looking at C vs C++
C++ and C are high-performance programming languages with widespread industrial applications. Let's explore some of these applications.
System Programming
C++ is widely used in system programming to develop operating systems, device drivers, and network applications. C, on the other hand, is commonly used for low-level system programming tasks such as memory allocation and handling.
Embedded Systems
Embedded systems are specialized computer systems designed to perform specific functions. C is the preferred language for embedded systems due to its small size, speed, and efficiency in memory usage. C++ is also used in embedded systems development, especially when object-oriented programming is required.
Game Development
C++ is a popular language for game development due to its performance and efficiency. Many game engines, including the popular Unreal Engine, are written in C++. C is also used in game development, particularly in console game development and for lower-level programming tasks.
Financial Applications
Both C and C++ are used extensively in the finance industry for developing high-frequency trading systems, accounting software, and risk management applications. In finance, speed and accuracy are critical, and both languages are known for their fast execution times and low memory usage.
Scientific Computing
C++ is used in scientific computing for developing numerical simulations, data analysis, and visualization applications. C++'s ability to handle complex mathematical operations and its compatibility with other programming languages make it an excellent choice for scientific computing. C is also used in scientific computing, particularly in developing system-level software for parallel computing architectures.
Concluding, we have examinedthe distinct characteristics and uses of C vs C++. It outlines that C is streamlined and well-suited for systems programming, while C++ offers object-oriented features, making it versatile for game development, system software, and scientific applications.
Both languages boast efficient performance and portability, though C++ has a richer standard library. The decision between using C or C++ hinges on the project’s complexity and the programmer’s preference. Despite the technological advances, both C and C++ retain their significance in the programming community,