We touch on Java Concurrency in our latest piece. Get hands-on with our practical code examples and prepare to revolutionize your Java applications.
More...
As an experienced Java developer, I've found that mastering the art of high-performance coding is an ongoing journey, especially with the evolution of Java's concurrency capabilities. Much like we discussed in a previous piece regarding concurrency vs parallelism in Python, similar, yet more advanced, principles apply to Java today. By understanding and harnessing concurrency in Java, you can truly transform your applications in terms of performance and responsiveness.
Understanding Concurrency
My journey into concurrent programming began years ago, with a web crawler application project. It was painstakingly slow, processing web pages in a sequential manner. Realizing the potential for parallelization, I decided to process multiple pages at once. This was my first brush with concurrency, a concept that's reshaped the way we approach programming.
What is concurrency?
Concurrency allows multiple parts of a program, or even the entire program, to run simultaneously. This is especially advantageous with modern multi-core CPUs, enabling improved throughput and interactivity. Implementing this in the web crawler led to a dramatic improvement in performance, showcasing the impact of concurrency in Java.
The Nitty-Gritty of Java Concurrency
Java's primary concurrency unit is the Thread. Each thread is a lightweight process with its own call stack but can access shared data of other threads within the same process. But, as we know, with great power comes great responsibility, and the basic threading capabilities are often insufficient for complex concurrent applications. Here's where Java's Concurrency Utilities fill the gap.
Java Concurrency Utilities provide a set of high-performance, thread-safe building blocks for creating concurrent applications or classes. This toolkit includes Java frameworks like the Executor framework, synchronizers, concurrent collections, locks, atomic variables, and the Fork/Join framework. It makes writing concurrent code much simpler, alleviating the need to construct everything from scratch.
Executor Framework
Once, I worked on an application involving heavy I/O operations. The application was creating new threads for each task, leading to a performance issue due to the overhead of thread creation and teardown. The solution? The Executor framework.
This framework abstracts the complexities of thread management, providing a straightforward way to manage and control task execution in a multithreaded environment. The Executor, ExecutorService, and ScheduledExecutorService interfaces, part of the java.util.concurrent package, facilitate this task execution management.
As of 2023, the CompletionService interface is gaining recognition for handling multiple Future objects easily. It allows you to decouple the invocation of asynchronous tasks and processing their results, enhancing flexibility and efficiency.
Below is an example of how to create a fixed thread pool with the Executor framework:
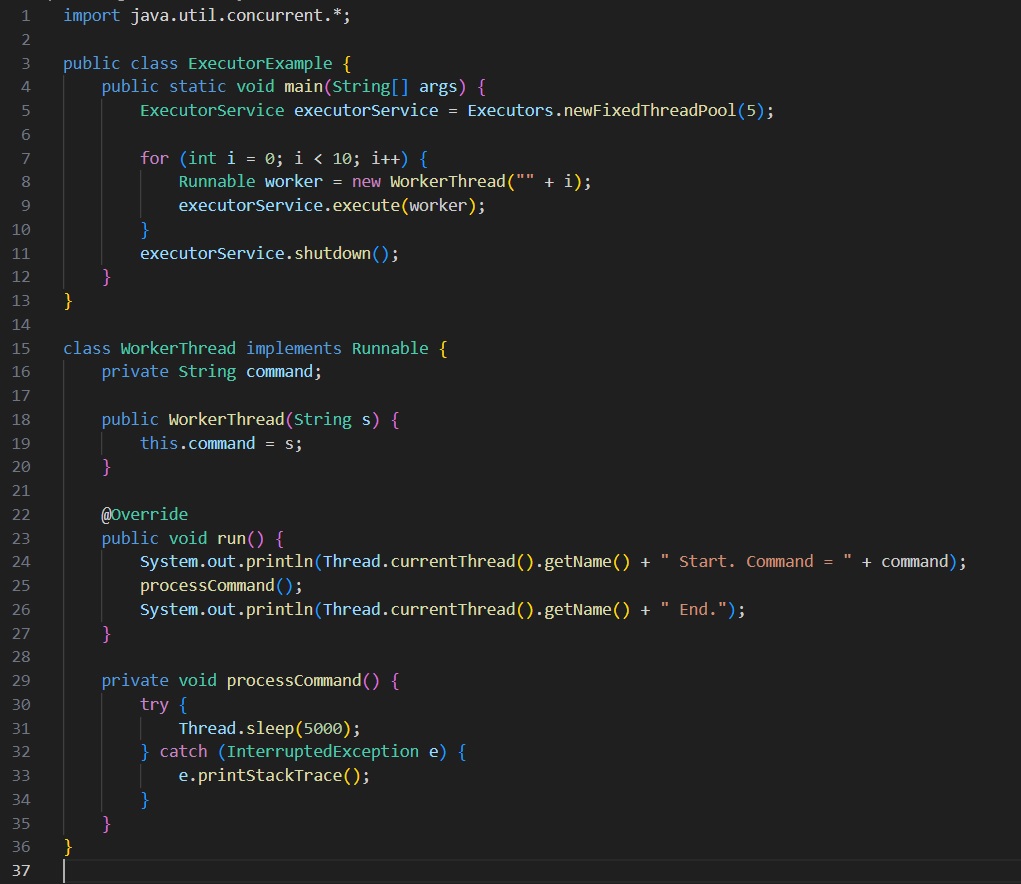
Synchronizers and Concurrent Collections
Next up are synchronizers—objects that control the flow of threads based on their state. Examples include CountDownLatch, Semaphore, and CyclicBarrier. I remember using a Semaphore to limit the number of threads accessing a specific resource.
Meanwhile, Concurrent Collections are thread-safe alternatives to data structures in the Java Collections framework. These include ConcurrentHashMap, ConcurrentLinkedQueue, and CopyOnWriteArrayList. They offer better scalability than regular collections by allowing concurrent access.
Java 9 introduced a few additions to this list, such as ConcurrentHashMap.compute and ConcurrentHashMap.computeIfAbsent, which allow atomically updating the value for a specific key.
Here's an example of using a ConcurrentHashMap:
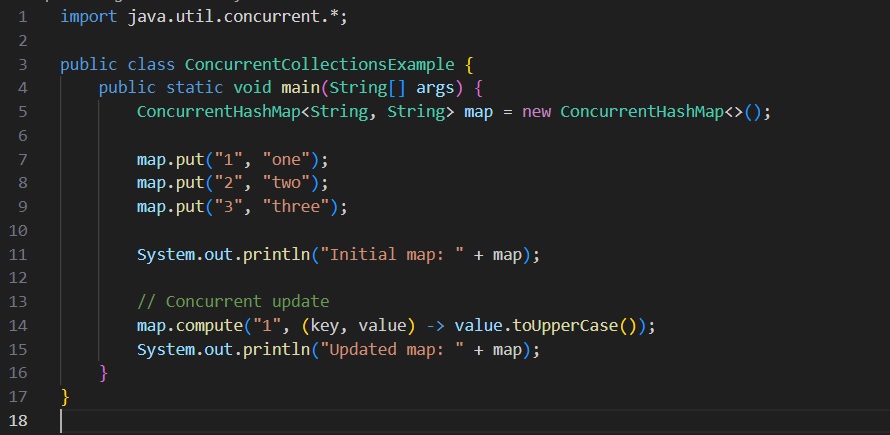
Let's look at how to use a CountDownLatch as a synchronizer. In this example, we'll create three worker threads. The main thread will wait until all worker threads finish their execution:
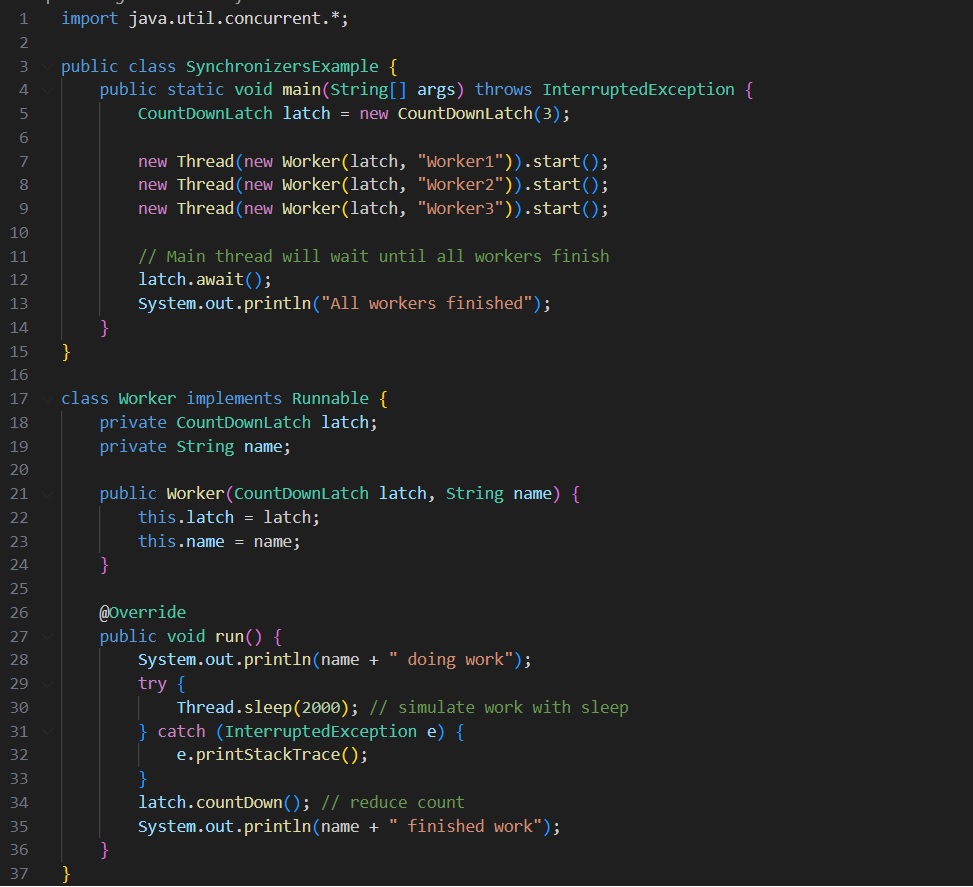
The Power of Virtual Threads
The evolution of Java concurrency has been remarkable. Java's Project Loom, for instance, introduced the concept of virtual threads (also known as fibers), potentially revolutionizing concurrency in Java. Virtual threads are lightweight and more efficient than regular threads, enabling more concurrent tasks without significant impact on system resources.
In 2023, with the evolution of Project Loom, developers have the added advantage of structured concurrency. This allows you to group and manage related tasks more effectively, preventing orphaned tasks and promoting cleaner, more understandable code. Virtual threads have become a more efficient alternative to traditional heavyweight OS threads, making Java a formidable language for writing concurrent applications.
Here's an example of creating a virtual thread:
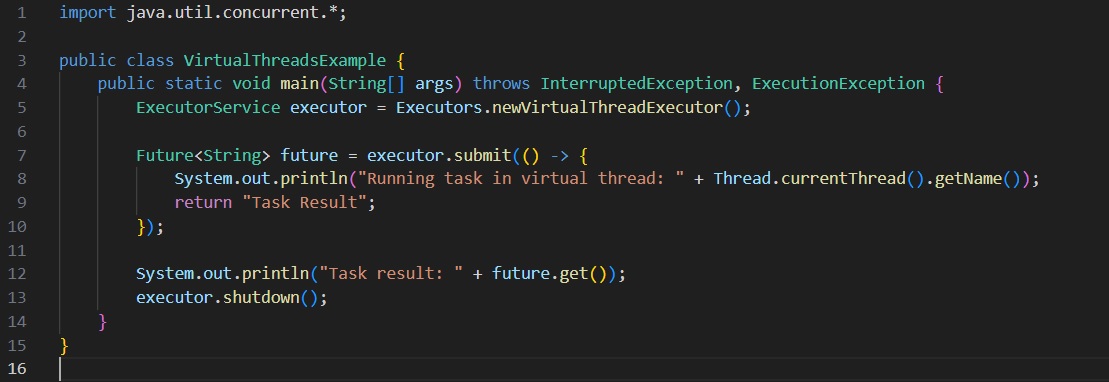
Conclusion
The landscape of concurrent programming in Java, with the evolution of Java Concurrency Utilities and advancements in Project Loom, is vibrant and ever-changing. With careful design and utilization of the right tools, it's possible to create applications that excel in performance and responsiveness.
In case you need experienced Java Developers
Our developers bring passion, innovation, and a constant drive to learn. Inject your team with the energy to create outstanding applications. Let's redefine performance and responsiveness together. Take advantage of our staffing services to complete your project with our...
Note
Reflecting on my journey with Java concurrency, from solving the performance issues of the web crawler to developing complex multithreaded applications, I'm grateful for Java's powerful concurrency tools. I invite you to explore the world of concurrent programming in Java, knowing that there's always something new to learn. Happy coding!