We feel like It's time to enhance your programming skills by mastering advanced techniques and strategies for variable usage.
More...
Variables is crucial for efficient coding. They help us manage and organize data, improve code readability, and enhance program flexibility. We can avoid repetitive calculations and optimize the processing power of our programs.
When aiming at efficient coding, one needs to sit down and learn the essentials of variables in programming.
As of right now, we will explore variable definitions, different types of variables, variable scope, and their importance in coding. Read through and you will have a solid understanding of variables and how to use them effectively in your programming endeavors.
Key Takeaways
What are Variables in Programming?
A variable is a designated area of memory used in programming to store a value. It serves as a placeholder that can be assigned different values and used in calculations or logical processes. To define a variable, a specific data value is assigned using the assignment operator.
For example, the code "x = 10" assigns the value 10 to the variable x. Variables can have different data types, such as integers, floats, strings, and booleans, depending on the type of value they store. When defining variables, it is important to choose meaningful and unique names that improve code readability.
Check out our detailed posts on Variables in Java and Variables in Ruby.
Exploring Variable Types
Variables in programming can have different types, which determine the kind of data they can store. By choosing the appropriate variable type based on the data being stored, programmers can ensure proper data processing. Look at some of the common variable types:
Assigning and Using Variables
Assigning values to variables is a fundamental aspect of programming. By using the assignment operator, developers can store specific values in variables for later use.
Let's glance at an example:
x = 10
In this case, we are assigning the value 10 to the variable x. It is important to assign meaningful initial values to variables that accurately represent the data they will store.
Once values have been assigned to variables, they can be used in various operations, manipulations, and control flow processes throughout a program. Variables act as placeholders for data, allowing developers to conveniently store and retrieve values when needed.
Here are some common use cases for variables in programming:
- 1Performing mathematical calculations
- 2Manipulating strings
- 3Storing user input
- 4Controlling program flow with conditionals and loops
Let's take a peek at how variables can improve a program's overall performance in the table below:
Without Variables | With Variables |
---|---|
10 + 10 + 10 + 10 | x = 10 |
Duplicate calculations | Reuse variable x |
More processing power required | Optimized processing |
Variable Declaration in Different Programming Languages
When it comes to declaring variables in programming, the syntax can vary across different languages. For sure, exploring how variable declaration works in popular high level programming languages like C/C++, Python, Java, JavaScript, and PHP is recommended!
C/C++
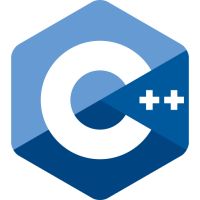
In C/C++, variables are declared using specific keywords followed by the variable name. For example, to declare an integer variable named "age", we would use the "int" keyword:
int age;
Similarly, the "char", "float", and "bool" keywords are used to declare character, floating-point, and boolean variables, respectively.
Python
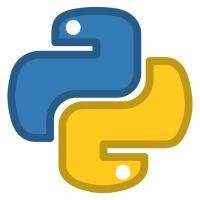
In Python, variable declaration is more straightforward. We can directly assign a value to a variable using the assignment operator. For example, to declare an integer variable named "count" and assign it a value of 10, we would write:
count = 10
Java
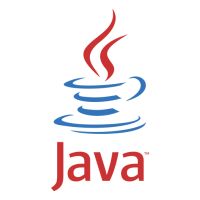
Java follows a syntax similar to C/C++. Variables are declared using keywords followed by the variable name. For example, to declare an integer variable named "score" in Java, we would use the "int" keyword:
int score;
JavaScript
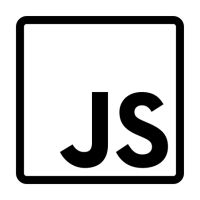
In JavaScript, the "var" keyword is used for variable declaration. For example, to declare a string variable named "name" in JavaScript, we would write:
var name;
PHP
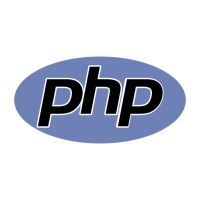
Similar to JavaScript, PHP also uses the "var" keyword for variable declaration. For instance, to declare a floating-point variable named "price" in PHP, we would use:
var price;
Variable declaration syntax in the chosen programming language is crucial for correctly declaring and using variables in your code. Let's take a closer look at variable scope in the next section.
For those interested in a career-focused approach to learning these languages, explore our Java Developer Roadmap, Python Developer Roadmap, and Software Developer Roadmap for comprehensive guidance.
Programming Language | Variable Declaration Syntax |
---|---|
C/C++ | int variableName; |
Python | variableName = value; |
Java | dataType variableName; |
JavaScript | var variableName; |
PHP | var variableName; |
Exploring Variable Scope
In programming, variables can have different scopes that define their accessibility within a program. Let's move on to the different types of variable scopes:
Local Variables
Local variables are only accessible within the block of code in which they are defined. They are typically declared within functions or code blocks and have a limited scope. Once the block of code is completed, local variables are no longer accessible. Using local variables helps keep code modular and prevents unintended conflicts with other variables in the program.
Global Variables
Global variables, on the other hand, can be accessed from anywhere within the program. They are not limited to a specific block of code and can be used across functions and code blocks. However, overusing global variables can make code harder to debug and maintain, as they can be modified by any part of the program.
Enclosed Variables
Enclosed variables are defined in outer functions or code blocks and are accessible by inner functions. They allow inner functions to access and use the values stored in the enclosed variables. This concept, known as "closure," enables powerful programming techniques like nested functions and function factories.
Built-in Variables
Built-in variables are part of the programming language's scope and are always accessible without needing any special declarations. They are pre-defined by the language itself and provide useful information or functionality. Examples of built-in variables include sys.argv in Python, which stores command-line arguments, or window.innerWidth in JavaScript, which gives the width of the browser window.
A well defined variable scope is essential for writing clean and efficient code. It helps prevent naming conflicts and allows for better organization of code. Minimizing the use of global variables, leveraging enclosed variables, and making use of built-in variables, programmers can create more robust and maintainable software.
Variable Scope Type | Description |
---|---|
Local Variables | Declared within a block of code and accessible only within that block. |
Global Variables | Accessible from anywhere within the program. |
Enclosed Variables | Defined in outer functions or code blocks, accessible by inner functions. |
Built-in Variables | Part of the programming language's scope, always accessible. |
Gain further insight into programming languages by reading about Python Libraries and comparing Java vs Python in terms of how they handle variable scopes and other features.
Importance of Variables in Programming
They are essential components that enable developers to store, manipulate, and reuse data with ease.
Improved Code Readability
Using variables in coding enhances code readability by providing meaningful names to represent data values. Instead of using hard-coded values directly in the code, variables act as placeholders that give context and clarity to the purpose of the stored data. This practice makes it easier for programmers to understand and maintain the code, as well as for other developers to collaborate and work with the code in the future.
Reduced Duplication and Increased Flexibility
Variables enable developers to avoid duplication of code by storing values that need to be reused multiple times. Instead of repeating the same data or calculations throughout the code, programmers can assign the value to a variable and reference it whenever needed. This not only reduces the chances of errors or inconsistencies but also makes it easier to modify the value in a single place, providing flexibility and saving time during development and maintenance.
Better Program Control and Flow
Variables allow programmers to control the flow of their programs by storing and manipulating data dynamically. By assigning values to variables, developers can perform calculations, make logical decisions based on conditions, and implement loops or iterations. This control over program flow increases the versatility and functionality of the code, enabling developers to create more interactive and responsive applications.
Efficiency and Performance Optimization
The use of variables in programming contributes to efficient and optimized code. By assigning values to variables, developers can avoid repetitive calculations or data processing, reducing the computational overhead. This optimization improves the overall performance of the program, making it faster and more resource-efficient. Variables also allow for better memory management, as they enable programmers to allocate and deallocate memory dynamically, optimizing the usage of system resources.
The benefits of variables in programming can be summarized as follows:
- 1Improved code readability
- 2Reduced duplication and increased flexibility
- 3Better program control and flow
- 4Efficiency and performance optimization
For those preparing for interviews or seeking to test their knowledge, our posts on Python Interview Questions and Answers and Java Interview Questions and Answers can be incredibly helpful.
Real-World Usage of Variables in Programming
The Music Playlist Analogy
To conceptualize variables in programming, consider the analogy of creating a music playlist. In this scenario, each song in the playlist represents a variable. Like variables, songs have different attributes: title, artist, duration, and genre. These attributes are akin to the data types in programming.
Imagine you're curating a playlist for a party. You select songs based on the mood, the time of the event, and the preferences of your guests. Similarly, in programming, variables are selected and assigned values based on the needs of the program. Just as you might update your playlist by adding, removing, or rearranging songs, in programming, variables can be reassigned, updated, or reordered as needed.
During the party, you might adjust the playlist—skipping a song, repeating a favorite, or adding a new track based on the audience's reaction. In programming, this is similar to variables being manipulated during the execution of a program, adapting to user input, or changing conditions.
In essence, variables in programming are like songs in a playlist: they can be individually distinct, reordered, and manipulated to achieve the desired outcome—in this case, the success of the party, or the effective functioning of a program.
A Simple Code Snippet with Variables
We will focus on a simple code snippet to demonstrate the usage of variables. For this example, we will use the Python programming language.
Code Snippet:
# Variable declaration
x = 5
# Using the variable in code
y = x + 3
# Printing the value of the variable
print(y)
In this code snippet, we declare a variable x and assign it the value of 5. Then, we use this variable to perform a calculation and store the result in the variable y. Finally, we print the value of y, which is 8 in this case.
This code snippet highlights how variables can be declared, assigned values, and utilized in code to perform operations. It showcases the practical application of variables in programming and how they can be used to store and manipulate data.
Conclusion
Variables are a critical aspect of programming that significantly contribute to efficient and effective code development. By enabling developers to store, manipulate, and reuse data, variables enhance code readability and scalability.
Mastery of variables is paramount to becoming a skilled programmer. With a firm grasp on variables, developers can unlock their full coding potential and create robust and flexible solutions.
FAQ
What are variables in programming?
Variables in programming are designated areas of memory used to store values. They serve as placeholders that can be assigned different values and used in calculations or logical processes.
How are variables defined in programming?
Variables are defined by providing a specific data value to store using the assignment operator. For example, "x = 10" assigns the value 10 to the variable x.
What are the different types of variables in programming?
There are several types of variables in programming, including integer variables for whole numbers, floating-point variables for numbers with decimal points, string variables for character sequences, and boolean variables for logical values.
How do I assign values to variables and use them in programming?
Values can be assigned to variables using the assignment operator. For example, "x = 10" assigns the value 10 to the variable x. Variables can then be used in operations, calculations, and control flow within the program.
How are variables declared in different programming languages?
Variable declaration syntax can vary across programming languages. In C/C++, variables are declared using keywords like "int", "char", "float", or "bool". In Python, variables are declared by assigning a value to a name directly. Java, JavaScript, and PHP use similar syntax to C/C++. Each language has its own rules for variable declaration.
What is variable scope in programming?
Variable scope determines where a variable can be accessed within a program. Local variables are only accessible within the block of code where they are defined, while global variables can be accessed from anywhere within the program. Enclosed variables are accessible by inner functions and built-in variables are always accessible.
Why are variables important in programming?
Variables are important in programming because they enable developers to store, manipulate, and reuse data efficiently. They improve code readability, reduce duplication, and make programs more flexible and scalable.
Can you provide a simple code snippet that demonstrates the usage of variables?
Certainly! Here's an example in Python:
x = 10
print(x)
This code snippet declares a variable named x and assigns the value 10 to it. The stored value is then printed to the console.
Why are variables important in programming?
Variables are important in programming as they allow developers to store, manipulate, and reuse data efficiently. They improve code readability, reduce duplication, and make programs more flexible and scalable.